edge filters
References :
- Done Reading
- Reading
- https://nptel.ac.in/courses/106/106/106106224/ [IIT Madras Week 2 part 1]
- Cornell CV lecture on Edge
- https://szeliski.org/Book/ [Book Chapter 3]
- https://www.wikiwand.com/en/Sobel%5Foperator
- Joseph Redmon Lectures
- https://evergreenllc2020.medium.com/fundamentals-of-image-gradients-and-edge-detection-b093662ade1b
- To read:
Questions :
- Criteria for a good edge detector
- Good detection
- find all real edges, ignoring noise or other artifacts
- Good Localization
- detect edges as close as possible to true edges
- Single Response
- return one point only for each true edge point
- Good detection
from skimage.filters import roberts, sobel, scharr, prewitt, farid
roberts_img = roberts(img)
sobel_img = sobel(img)
scharr_img = scharr(img)
prewitt_img = prewitt(img)
farid_img = farid(img)
Robert, Scharr, Prewitt, Farid
![Figure 1: from nptel [IIT Madras] CV lecture slide Week 2 part 1](/blogs/ox-hugo/2021-05-30_15-35-13_screenshot.png)
Figure 1: from nptel [IIT Madras] CV lecture slide Week 2 part 1
The x-coordinate in figure ref:edge-filter-kernel is defined as increasing in the “right”-direction, and the y-coordinate is defined as increasing in the “down”-direction. At each point in the image, the resulting gradient approximations can be combined to give the gradient magnitude, using: [wikipedia]
\( {M} = \sqrt{ ({M}_{x})^{2} + ({M}_{y})^{2}} \)
Using this information, we can also calculate the gradient’s direction(orientation):
\( \Theta = \arctan{\frac{M_{y}}{M_{x}}} \)
where, for example, \(\Theta\) is 0 for a vertical edge which is lighter on the right side.
Sobel
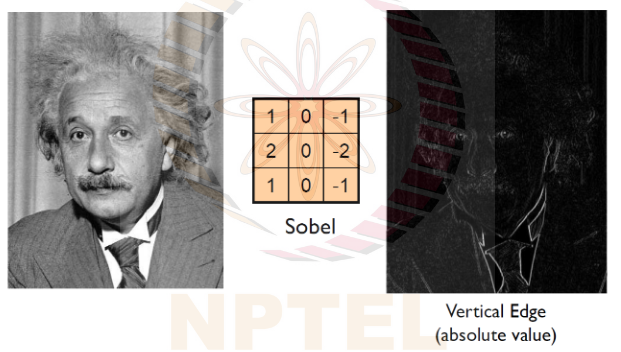
- Computes the image gradient in the x direction
![Figure 2: from nptel [IIT Madras] CV lecture slide Week 2 part 1](/blogs/ox-hugo/2021-05-30_15-44-40_screenshot.png)
Figure 2: from nptel [IIT Madras] CV lecture slide Week 2 part 1
- Computes the image gradient in the y direction
Canny edge
Algorithm
- Smooth image (only want “real” edges, not noise)
- Calculate gradient direction and magnitude
- Non-maximum suppression perpendicular to edge
- check if pixel is local maximum along gradient problem
- Threshold into strong, weak, no edge
- Define two thresholds: low and high
- hysteresis:
- Use the high threshold to start edge curves and the low threshold to continue them
- if gradient at pixel > ‘High’ = ’edge pixel’
- if gradient at pixel < ‘Low’ = ’non edge pixel’
- if gradient at pixel >= ‘Low’ and <= ‘High’ = ’edge pixel’ iff,
- it is connected to an ’edge pixel’ directly or via pixels between ‘Low’ and ‘High’
- Connect together components
- Tunable: Sigma, thresholds
- Canny edge pipeline
![Figure 3: from nptel [IIT Madras] CV lecture slide Week 2 part 1](/blogs/ox-hugo/2021-05-30_19-18-30_screenshot.png)
Figure 3: from nptel [IIT Madras] CV lecture slide Week 2 part 1
- Effect of \(\sigma\) in Cannyu Edge Detector
- The choice of σ (Gaussian kernel spread/size) depends on desired behavior
- large σ detects large-scale edges
- small σ detects fine edges
- The choice of σ (Gaussian kernel spread/size) depends on desired behavior
![Figure 4: from nptel [IIT Madras] CV lecture slide Week 2 part 1](/blogs/ox-hugo/2021-05-30_20-29-59_screenshot.png)
Figure 4: from nptel [IIT Madras] CV lecture slide Week 2 part 1
Python implementation
canny_edge = cv2.Canny(img, 50, 80)
# auto canny
sigma = 0.3
median = np.median(img)
lower = int(max(0, (1.0 - sigma) * median))
upper = int(min(255, (1.0 + sigma) * median))
auto_canny = cv2.Canny(img, lower, upper)